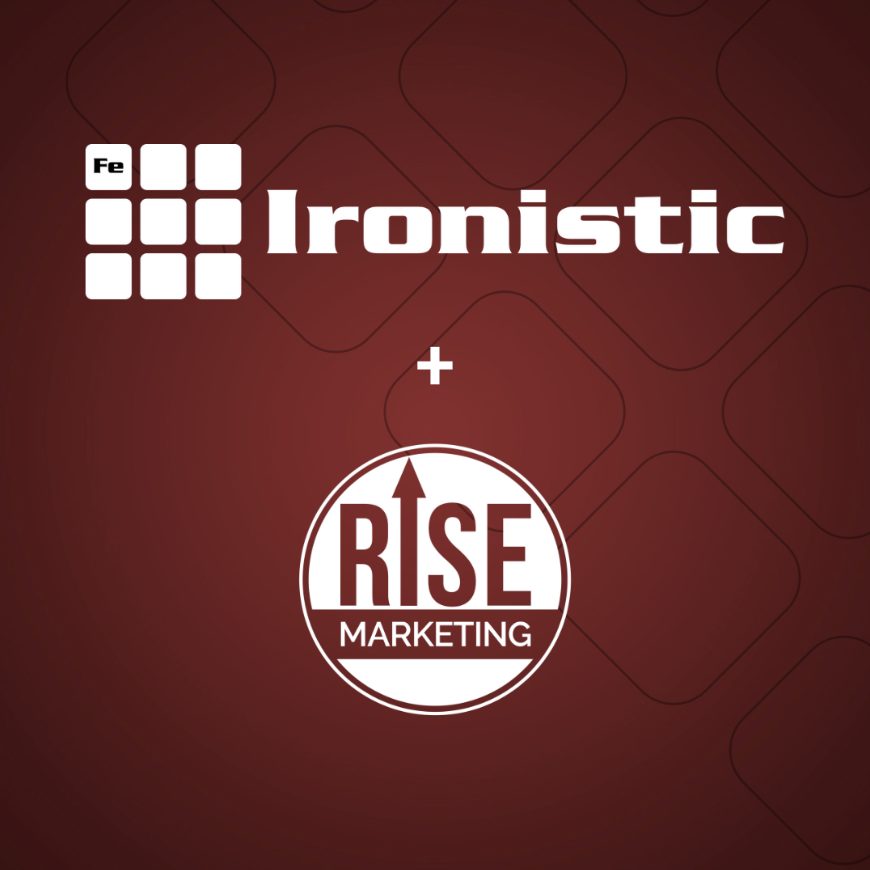
RISE Marketing Joins with Ironistic to Extend Marketing Communications Service Offering
Explore the difference between style guide vs. brand guide, what to include in each, and why you need both for…
Read More
PHP sessions are a great way to track and store information specific to a user’s session within a PHP application. In this article, I will show you how to use PHP sessions in a WordPress theme. In a standard PHP application, a session would be started using the session_start function at the very top of the PHP script. This may tempt you to open the header.php file in your WordPress theme and add something like the following to begin using sessions.
<?php session_start(); ?>
<!DOCTYPE html>
<head> ....
Though this would work, it is not the most efficient way to start a session in WordPress. WordPress provides an Actions API that we are able to attach custom functions to. That is the method we will be using in this example. We are going to add all the following code to the very top of our themes functions.php file.
1: We will use the init action provided by WordPress to handle starting a PHP session. First, we add the action that will call a function called start_session like this when WordPress first initiates.
add_action('init', 'start_session', 1);
2: Second we will create the start_session function. Notice the function is first checking to see if a session is already active before setting a new one using a function called session_id.
function start_session() {
if(!session_id()) {
session_start();
}
}
PHP provides a built-in function called session_destroy, that will handle clearing out all session data. However, when to call this function can be tricky to handle depending on the application. WordPress also provides a few ways for us to do just that in the Actions API.
1: In WordPress, we are going to need to clear out the session once a user has logged out or a new user has logged into the website. We will use two provided actions, wp_logout and wp_login, to call a function we will create called end_session();
add_action('wp_logout','end_session');
add_action('wp_login','end_session');
2: Second we will create the end_session function like this:
function end_session() {
session_destroy ();
}
We can use a custom hook to end a session anywhere within the theme by using the do_action function provided by WordPress to call the end_session function we created above.
1: In the functions.php file we would add the following.
add_action('end_session_action', 'end_session');
2: Add the following anywhere in the application you want to end the session.
do_action(
'end_session_action'
);
If done correctly your functions.php file should now look like this at the top.
add_action('init', 'start_session', 1);
function start_session() {
if(!session_id()) {
session_start();
}
}
add_action('wp_logout','end_session');
add_action('wp_login','end_session');
add_action('end_session_action','end_session');
function end_session() {
session_destroy ();
}
You can now add data to the global $_SESSION variable that will be accessible at any point within the application during a user session by accessing the $_SESSION variable. The variable is an array; below is an example of adding data to the session array.
$foo = 'Foo Data';
$_SESSION['foo'] = $foo;
Something to think about:
If you are building a scalable or load-balanced website, you may not want to use sessions. HTTP is Stateless and PHP SESSIONS are State-driven. Sessions are stored and handled by the server. Routing each session to the proper server requires a more complex configuration and creates a single point of failure for the users whose sessions are stored on that server. When possible, it is best to store session information in the client’s browser. Though it may not be extremely expensive for the server resources to query session objects, it is always wise to reduce overhead whenever possible.
Thank you for reading. I hope you find this article helpful and please comment below with any questions. Happy coding! 🙂
There are currently 17 responses.
April 12, 2021
This may have worked in the past but from what I heard, WordPress changed and won’t let you start a session anymore. Please correct me if I am wrong. I had to just session_start(); and it works fine but it doesn’t start for me if I use add_action(‘init’….. Also, by running session_start() , the site health complains loudly, it says…
A PHP session was created by a session_start() function call. This interferes with REST API and loopback requests. The session should be closed by session_write_close() before making any HTTP requests.
Also it causes a failure with REST API as it predicted above and gives this error.
The REST API is one way WordPress, and other applications, communicate with the server. One example is the block editor screen, which relies on this to display, and save, your posts and pages.
The REST API request failed due to an error.
Error: cURL error 28: Operation timed out after 10002 milliseconds with 0 bytes received (http_request_failed)
I must have session for the plugin I am building and I am going bald pulling my hair out over this issue.
June 1, 2021
Les F.
I just found some time to test this on a brand new WP installation and I am not running into the same issue you are seeing. The code is still working as expected on my end.
March 27, 2021
I use Sessions in one of the plugins I built for a client. I recently began getting this message in “Site Health Status”: “A PHP session was created by a session_start() function call. This interferes with REST API and loopback requests. The session should be closed by session_write_close() before making any HTTP requests.” In doing some more digging, it appears WordPress began integrating its REST API in Version 4.7 and had also integrated it into wp-admin by version 4.8.0. As far as I can tell from other reading, the REST API will not work with an open PHP Session — which seems to imply that the use of PHP sessions in WordPress is probably a thing of the past. Have I got this right?
At present cookies seem to be the only viable alternative. Is that your take?
August 18, 2020
Hi Nathan,
Sessions can be quite trick for beginners to understand so thank you for putting this together and helping educate poeple.
Great site you have here too!
Take care
Jamie
April 11, 2019
Nice tutorial. Thank you!
February 25, 2019
Please note that some of the add_action statements above have “smart quotes” (left single quote and right single quote). PHP won’t parse them correctly if people attempt to copy the code. Please ensure that proper single quotes are used so that if the code is pasted into a text document, it will parse correctly.
I believe the ‘init’ add_action is OK. The other three add_action statements seem to have left and right quotes.
February 25, 2019
This also has been updated. Was missing some
blocks.
February 25, 2019
Where you show the function start_session() the second time, the second right brace is missing. This will cause web sites not to function if any attempts to copy/paste the code.
February 25, 2019
You are correct. Thanks for letting us know. I have updated the posts and corrected it.
June 14, 2018
Thanks for the article. What I don’t quite get though is the session ending part. A session is meant to end when a user logs in?
So if user 1 logs in and doesn’t log out, user 1 session will end when user 2 logs in? How will that work in a private site where each visitor is a user?
November 7, 2017
Hello, nice tutorial explained very clearly, however, this procedure only works using the functions, whereas using OOP programming generates an error.
In particular I did a kind thing like:
class MyClass
{
public function __construct()
{
add_action( ‘init’, array( $this, ‘session_start’ ), 1 );
}
public function session_start()
{
if ( ! session_id() )
session_start();
}
}
but i get this error:
Warning
: call_user_func_array() expects parameter 1 to be a valid callback, function ‘megamall_compare_setup_plugin’ not found or invalid function name in
C:my_serverwp-includesclass-wp-hook.php
on line
298
August 24, 2017
Thank you!
August 10, 2017
This looks like it will work well. I’m converting an extensive Classic ASP website to a WordPress site and writing plugins to handle the admin functionality within the website. You mention in the last paragraph: “When possible, it is best to store session information in the client’s browser”….are you referring to the setCookie(cookie,cookieValue) call and $_COOKIE[‘cookie’] or to some other mechanism to make that happen?
October 4, 2016
It worked Great